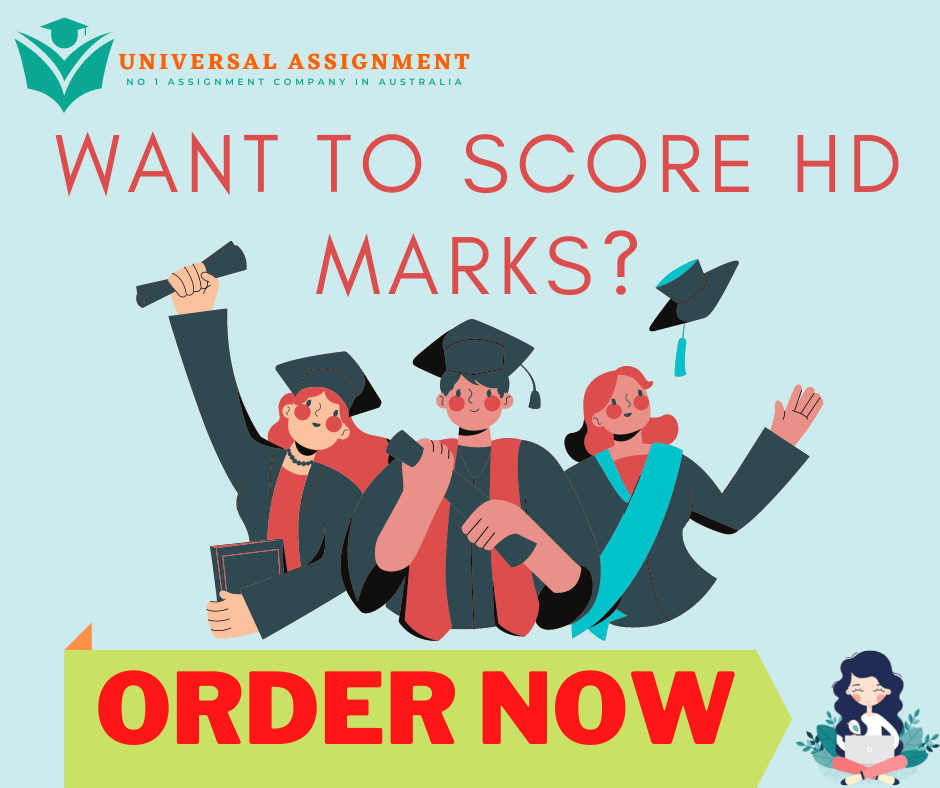
Note:
Add a slash before the nav routes in the _Layout.cshtml file otherwise you may get an exception when changing pages
- href=”/Home”>Home</a></li>
- href=”/Catalogue”>Products</a></li>
Also add a slash before the css and also the image paths at the top if you haven’t done this already
i.e. <link rel=”stylesheet” href=”/css/style.css”>
Product Details Page
- Add a ProductDetails() method to the catalogue controller
- Pass one parameter into the ProductDetails method which will be int productId
- We will set up the route for this in a soon to pass the product id to the controller
- Retrieve the product from the database. For the LINQ command use the code below (from Listing 8.25 which uses product id to fetch the product)
- Watch the case for the product id variable and database field in the method parameters and LINQ
Product product = repository.Products
.FirstOrDefault(p => p.ProductID == productId);
- Pass the product to the view
- Add a corresponding ProductDetails view to the catalogue view folder
- The model for the view will be of type Product
- Add the body (excluding header and footer) from the single-product.html template file provided and copy it into the view
- Populate the page using the product model that was passed in
- Don’t forget the image. Check the catalogue page for the format
- Also add a slash here before img
src=/img/product/@Model.Image …
Open from the Catalogue Page
- Next, we need to open the product details page when the product image is clicked in the catalogue page. For this we need to pass the product id of the image clicked
- We could set up a route to the product details method in startup.cs and pass the product id
app.UseEndpoints(endpoints => {
endpoints.MapControllerRoute(“ProductDetails”, “Product/{productID}”,
new { Controller = “Catalogue”, action = “ProductDetails” });
endpoints.MapDefaultControllerRoute();
});
- However, it is easy to use a tag helper in the Catalogue controller as we did before
- Note that the route name does not have to have any association with the controller and method names so you can name it anything meaningful
[Route(“Product/{productId}”)]
public IActionResult ProductDetails(int productId)
Add the link to the Catalogue Product Image
- Add a link around the product image in our partial view for the image click
- Use the route above and pass the product id in the route
<a href=”/product/@Model.ProductID”>
<img …>
</a>
Test
- The product details page should now open when an image is clicked and display the products details for that product
Cart
- You can follow Listing 8.13 to 9.6 as a guide for the cart
- However, the cart is something universal and is reasonably portable so you may copy the cart files from SportsStore. Still, you should check this against the text
- Copy the shopping cart class and the session cart classes from the Models folder
- Create the Infrastructure folder in the root
- Copy SessionExtensions.cs to this folder
- Create the Pages Folder
- From the Pages folder copy the _CartLayout.cshtml, _ViewImports.cs, _ViewStart.cs and Cart.cshtml and Cart.cshtml.cs files
- Be careful to change to your namespace
Startup.cs
- See Listing 8.13, Listing 8.21 and Listing 9.3 to update the startup configuration for Razor Pages, Sessions, HTTPContext and Cart as a service
Test
- Open the cart page using the URL below and check that the page is displayed
- Note that the cart will be empty
Add to Cart Button
- In the Product Details page, we wrap the quantity and Add to Cart button in a Form (based on Listing 8.21) so we can add items to the cart
- Above the product_count div, insert the code for the start of the form
<form asp-page=”/Cart” method=”post”>
<input type=”hidden” name=”productId” value=”@Model.ProductID”/>
- Below the submit button, close the form
<input type=”hidden” name=”returnUrl”
value=”/Catalogue />
</form>
Test
- Test the add to cart. It should add one item to the cart each time the Add to Cart button is pressed
- The remove item should delete an item from the cart
- The Continue Shopping button should return you to the Catalogue page
Note
- I have provided less guidance for the items below which may require you to do a little research
Cart Layout
- You will need to copy the main layout for the cart layout and alter as required
- You will need to remove the footer
- or add a margin on the top of the footer to create a gap below the buttons
Product Quantity
- The SportsStore cart page only added a single item per click, but we need to add the quantity from the spin control. Fortunately, the template markup has the value as a text input named quantity. For this you will need to modify the OnPost method
Test
- Test the add to cart. The quantity set should be added to the product quantity in the cart
Cart Nav
- Add the Cart icon button onClick event to open the cart
Cart Icon
- Change the number beside the cart icon in the header to reflect the total number of items in the cart. For this you can inject the Cart into both controllers and use the ViewBag or create a component as in the Sports Store.
Complete all other items in the specification and marking criteria.
- Note that you may change the text on the Sale button
