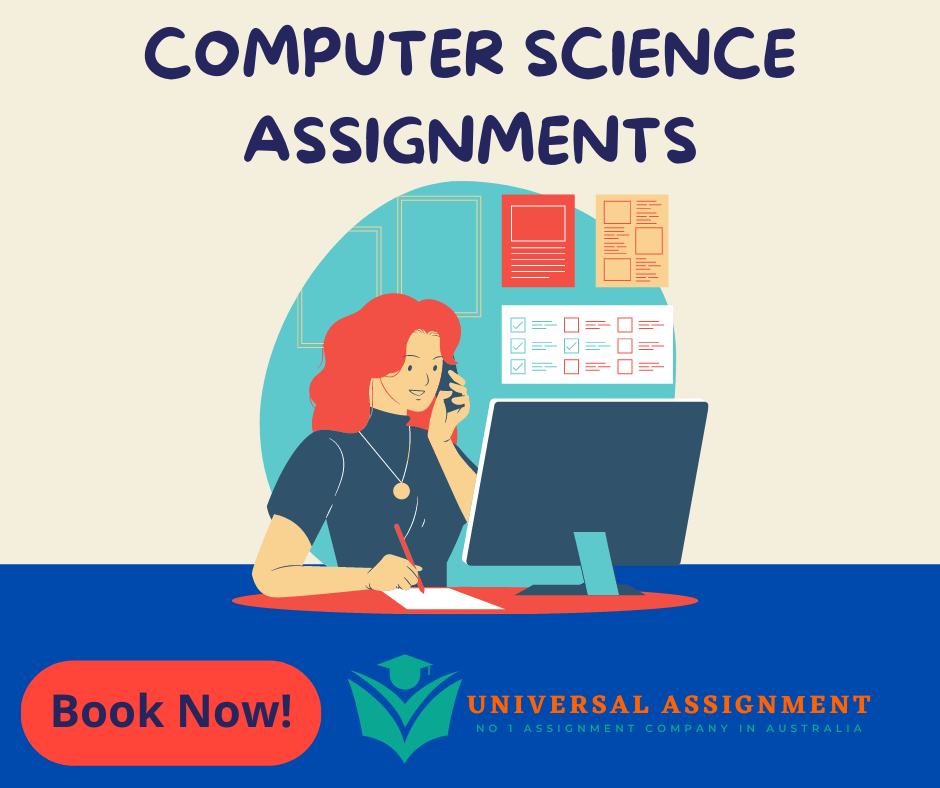
Question 1
In this exercise we’ll be using a combination of things we’ve worked on before, but now starting almost from scratch. No individual element here is new, so read the instructions carefully and break the task down into steps.
In the skeleton you are given a class Sequence
with very little code in it. It starts with an array called sequence
, and that’s about it.
Below the indicated comment you need to do the following (you may want to think about the order):
- Create a standard
main
method. In the main method you need to:- Create a
Scanner
object to be used to read things in
- Print a prompt to
"Enter the first double: "
, without a new line after it.
- Read a
double
in from the user and store it as the first element ofsequence
.
- Print a prompt to
"Enter the second double: "
, without a new line after it.
- Read a
double
in from the user and store it as the second element ofsequence
.
- Calculate a third
double
which is the seconddouble
divided by the firstdouble
, all multiplied by the seconddouble
.
- Store this third
double
as the third element ofsequence
.
- Print
"The first element was <x>, the second element was <y>, so the third element is <z>."
where<x>
,<y>
and<z>
are replaced by the values of the first, second and thirddoubles
respectively.
- Create a
Remember that computers aren’t clever, so note the formatting and punctuation, especially in the feedback of the tests.
Note also that part of the task is to use the array sequence
. Although you can print out the correct things without it, you cannot pass the task.
As an example, you have also been given the class AreaOfRectangle
, you do not need to change anything, it is just
Starter Code Question one
import java.util.Scanner;
public class Sequence {
static double [] sequence = {0, 0, 0};
// DO NOT CHANGE ANYTHING ABOVE THIS COMMENT LINE
//Create a main method
//Create a Scanner
//Prompt the user as per the description and read in a double.
//Prompt the user as per the description and read in another double.
//Calculate the third double.
//Print out the message about the sequence values.
//Don’t forget to put a closing brace at the end of the main method.
}
Question 2
In this exercise we’ll be using a slightly different combination of things we’ve worked on before, starting from scratch again. No individual element here is new, so read the instructions carefully and break the task down into steps.
In the skeleton you are given a class ThreeNumbers
which starts with an array called num
, and little else.
Below the indicated comment you need to do the following (you may want to think about the order):
- Create a standard
main
method. In the main method you need to:- Create a
Scanner
object to be used to read things in
- Print a prompt to
"Enter the first number: "
, without a new line after it.
- Read an
int
in from the user and store it as the first element ofnum
.
- Print a prompt to
"Enter the second number: "
, without a new line after it.
- Read an
int
in from the user and store it as the second element ofnum
.
- Print a prompt to
"Enter the third number: "
, without a new line after it.
- Read an
int
in from the user and store it as the third element ofnum
.
- Print
"The sum of the three numbers is <sum>."
, with a new line after it, where<sum>
is replaced by the actual sum of the elements ofnum
.
- Print
"The average of the three numbers is <avg>."
, with a new line after it, where<avg>
is replaced by the actual average (rounded down, so you can use integer division) of the the elements ofnum
.
- Create a
Remember that computers aren’t clever, so note the formatting and punctuation, especially in the feedback of the tests.
Note also that part of the task is to use the array num
. Although you can print out the correct things without it, you cannot pass the task.
Question 2 Starter Code:
import java.util.Scanner;
/*
class ThreeNumbers
static int [] num = {0, 0, 0};
// DO NOT CHANGE ANYTHING ABOVE THIS COMMENT LINE
//Create a main method
//Create a Scanner
//Prompt the user as per the description and read in an int.
//Prompt the user as per the description and read in another int.
//Prompt the user as per the description and read in the third int.
//Calculate the sum.
//Calculate the average.
//Print out the messages about the sum and average.
//Don’t forget to put a closing brace at the end of the main method.
}
Question 3
In this exercise the goal is to implement the Bubble Sort algorithm on a small array. If you are comfortable with loops and arrays, you can solve this by implementing the full algorithms. If you are not, you can “unroll” the loop and implement each step by hand by hard coding each pass through the array as a sequence of explicit checks and potential swaps.
The skeleton contains two methods:
- A
main
method which will allow you to do your own testing, and give a working example. - A
bubbleSort
method where you’ll do the actual implementation of the sorting algorithm.
The bubbleSort
method takes in an int[]
called data
. This is the array that you are to sort. You are guaranteed for this exercise that data
will have length 4. At the end of the method you should return data;
. This line has already been added, so you can just leave it as is.
For the tests to ensure you are correctly implementing a Bubble Sort (and not some other sort, or using a library), you must print out the array using printArray(data)
every time you swap elements in the array. You should not print it out any other time in the bubbleSort
method.
Question 3 – Starter Code
import java.util.Arrays;
public class BubbleSort {
//Don’t touch this method!
private static void printArray(int[] a) {
System.out.println(Arrays.toString(a));
}
//Complete this method.
public static int[] bubbleSort(int[] data) {
//Implement a Bubble Sort on data here!
return data;
}
//You can mess around in the main method
//as you wish. As long as it compiles,
//it won’t affect the testing.
public static void main(String[] args) {
int[] testData = {45, 93, 33, 55};
System.out.println(“Sorting.”);
testData = bubbleSort(testData);
System.out.println(“After sorting the array is: “);
printArray(testData);
}
}
Question 4 :
In this exercise the goal is to implement the Selection Sort algorithm on a small array, in a similar manner to the Bubble Sort required exercise. If you are comfortable with loops and arrays, you can solve this by implementing the full algorithms. If you are not, you can “unroll” the loop and implement each step by hand by hard coding each pass through the array as a sequence of explicit checks and potential swaps.
The skeleton contains two methods:
- A
main
method which will allow you to do your own testing, and give a working example. - A
selectionSort
method where you’ll do the actual implementation of the sorting algorithm.
The selectionSort
method takes in an int[]
called data
. This is the array that you are to sort. You are guaranteed for this exercise that data
will have length 4. At the end of the method you should return data;
. This line has already been added, so you can just leave it as is.
For the tests to ensure you are correctly implementing a Selection Sort (and not some other sort, or using a library), you must print out the array using printArray(data)
every time you swap elements in the array. You should not print it out any other time in the selectionSort
method. For consistency in which version of Selection Sort is implemented:
- Stop the sort once the unsorted area is only one element.
- Don’t swap if the minimum element is the first element in the unsorted area (so no print out in this case).
Question 4 Starter Code
import java.util.Arrays;
public class SelectionSort {
//Don’t touch this method!
private static void printArray(int[] a) {
System.out.println(Arrays.toString(a));
}
//Complete this method.
public static int[] selectionSort(int[] data) {
//Implement a Selection Sort on data here!
return data;
}
//You can mess around in the main method
//as you wish. As long as it compiles,
//it won’t affect the testing.
public static void main(String[] args) {
int[] testData = {45, 93, 33, 55};
System.out.println(“Sorting.”);
testData = selectionSort(testData);
System.out.println(“After sorting the array is: “);
printArray(testData);
}
}
Question 5
This exercise is similar to the “Calling Methods” exercise, but flipped. Here we will be filling out methods so that they function as specified. The code here is also a little “simpler”, in that it is contained in a single class, and everything is static
, so we don’t need to deal with creating objects just yet.
In the skeleton you have a class called BankAccountStatic
. The class has three data members accountNumber
, accountName
and balance
. You do not need to do anything to these declarations.
It contains two completed methods: a main
method which runs at least one example of each method, you can change the main as much as you like, as long as it compiles, it will not affect the marking; a method called withdraw
, this method should serve as an example for the methods you have to complete, although the methods you have to complete are typically much simpler, do not change this method.
Your task is to complete these six methods:
getAccountNumber
setAccountNumber
getAccountName
setAccountName
getBalance
deposit
Each of this represents a method that either accesses or changes one of the three data members, as suggested by the name. You should read the comment block associated with each method, as it describes what needs to be complete for each method.
Question 5 Starter Code
/**
* This “BankAccount” class is a simple introduction to
* methods and variables / fields / private data members.
*
/*
* HINT: You should NOT need to memorise much to complete
* this skeleton. The type of the variables
* “accountNumber”, “accountName” and “balance”
* (i.e. int, String and double respectively)
* provide useful information.
*/
public class BankAccountStatic
{
/*
* The variables follow. These are also
* called “fields” or “?”private data members”.
*/
private static int accountNumber = 0;
private static String accountName = “no name”;
private static double balance; // eg. 1.27 means $1.27
public static void main(String[] args) {
System.out.println(“The initial value for account number is ” + accountNumber + “. Look at line 24.”);
System.out.println(“The initial value for account name is ” + accountName + “. Look at line 26.”);
System.out.println(“The initial value for balance is ” + balance + “. Look at line 28.”);
BankAccountStatic.setAccountNumber(12345678);
BankAccountStatic.setAccountName(“Samantha”);
//the above two lines should update the values stored in accountNumber and accountName to the given value. Let’s test it:
System.out.println(“The new account number is 12345678. Your program’s new account number is ” + accountNumber +”. If these two numbers don’t match then something is not right…”);
System.out.println(“The new account name is Samantha. Your program’s new account name is ” + accountName+”. If these two names don’t match then something is not right…”);
//lets put some money in the account
BankAccountStatic.deposit(3.70);
//the new balance should be 3.70. Let’s check it:
System.out.println(“New balance should be 3.70. Your program’s new balance is ” + balance+”. If these two numbers don’t match then something is not right…”);
//let’s put some more money in our bank account:
BankAccountStatic.deposit(100.00);
//the new balance should be 103.70. Let’s check it:
System.out.println(“New balance should be 103.70. Your program’s new balance is ” + balance+”. If these two numbers don’t match then something is not right…”);
//let’s withdraw some money now. the new balance should be 93.20. Let’s check it:
System.out.println(“After this line, the new balance should be 93.20. Your program’s balance after calling withdraw(10.50) is ” + BankAccountStatic.withdraw(10.50) + “. If these two numbers don’t match then something is not right…”);
//let’s attempt to withdraw more than what we have in the account. The result of print statement should be zero:
System.out.println(“Attempting to withdraw 1000.00 dollars from this bank accoutn should return a -1.0. Your program’s return value after calling withdraw(1000.00) is ” + BankAccountStatic.withdraw(1000.00) + “. If these two numbers don’t match then something is not right…”);
}
/**
* This method needs to be completed. It needs:
* – a proper return type
* – a proper list of parameters (maybe empty)
* – code to actually do what it should
*/
public static returntype getAccountNumber(parametersIfAny)
{
add whatever code is required here in the method body
}
/**
* This method needs to be completed. It needs:
* – a proper return type
* – a proper list of parameters (maybe empty)
* – code to actually do what it should
*/
public static returntype setAccountNumber(parametersIfAny)
{
add whatever code is required here in the method body
}
/**
* This method needs to be completed. It needs:
* – a proper return type
* – a proper list of parameters (maybe empty)
* – code to actually do what it should
*/
public static returntype getAccountName(parametersIfAny)
{
add whatever code is required here in the method body
}
/**
* This method needs to be completed. It needs:
* – a proper return type
* – a proper list of parameters (maybe empty)
* – code to actually do what it should
*/
public static returntype setAccountName(parametersIfAny)
{
add whatever code is required here in the method body
}
/**
* This method needs to be completed. It needs:
* – a proper return type
* – a proper list of parameters (maybe empty)
* – code to actually do what it should
*/
public static double getBalance(parametersIfAny)
{
add whatever code is required here in the method body
}
/**
* This method needs to be completed. It needs:
* – a proper return type
* – a proper list of parameters (maybe empty)
* – code to actually do what it should
*/
public static returntype deposit(parametersIfAny)
{
add whatever code is required here in the method body
}
/**
* @param amount To be subtracted from the balance
*
* @return the updated account balance or -1.0 if the withdrawal is refused
*
* The withdrawal is refused when the withdrawal would
* have resulted in a negative balance.
*
* Note: This method “withdraw” has been provided in
* its entirety. You do NOT have to make any
* changes to it.
*/
public static double withdraw(double amount)
{
if ( balance >= amount )
{
balance = balance – amount;
return balance;
}
else
return -1.0;
}
} // class BankAccountStatic
Question 6
Here will we again build some methods, but in a slightly different context, to help build the programming links between the code and your conception of it, rather than just understanding how a bank account should work.
In the skeleton you have a class called SummerStatic
. The class has two data members sum
and count
. You do not need to do anything to these declarations. The purpose of the class is to receive numbers one by one, and update the current sum
and count
, and thus be able to report on the sum, average and count. The sequence can also be reset if desired.
It contains one completed method: a main
method which runs at least one example of each method, you can change the main as much as you like, as long as it compiles, it will not affect the marking.
Your task is to complete the following six methods:
putNumber
reset
- a different
reset
getSum
getCount
getAverage
You should read the description of each method in the associated comment block carefully as it describes the purpose of each method, and what needs to be completed for the method
Question 6 Starter Code
/**
* This “Summer” class is a simple introduction to
* methods and variables / fields / private data members.
*
public class SummerStatic
{
/*
* The variables follow. These are also
* called “fields” or “private data members”.
*/
private static int sum; // sum of all integers received
private static int count; // number of integers received
public static void main(String [] args)
{
/*
* Note: This method “main” has been provided in
* its entirety. You do NOT have to make any
* changes to it.
*
* You could use this “main” method to test your code.
*/
System.out.println(“First we call reset so that th evalues of sum and count are set to zero.”);
reset();
System.out.println(“Now we assign numbers 17 and 1.”);
putNumber(17);
putNumber(1);
System.out.println(“Now we call getCount, getSum and getAverage in three print statements to check the values.”);
System.out.println(“count should be 2, sum should be 18 and average should be 9.00:”);
System.out.println(“Now we call getCount, getSum and getAverage in three print statements to check the values.”);
System.out.println(“”);
System.out.println(“”);
System.out.print(“count = ” + getCount() + ” “);
System.out.print(“sum = ” + getSum() + ” “);
System.out.println(“average = ” + getAverage());
System.out.println(“”);
System.out.println(“”);
System.out.println(“”);
System.out.println(“”);
System.out.println(“”);
// Repeat for a second set of numbers
System.out.println(“We run the program again, this time we start by calling reset(3)”);
reset(3);
System.out.println(“Now we assign numbers 5 and 7.”);
putNumber(5);
putNumber(7);
System.out.println(“Now we call getCount, getSum and getAverage in three print statements to check the values.”);
System.out.println(“count should be 3, sum should be 15 and average should be 5.00:”);
System.out.println(“”);
System.out.println(“”);
System.out.print(“count = ” + getCount() + ” “);
System.out.print(“sum = ” + getSum() + ” “);
System.out.println(“average = ” + getAverage());
} // method main
/**
* Receives and processes a new number in the series
* This method needs to be completed. It needs:
* – a proper return type
* – a proper list of parameters (maybe empty)
* – code to actually do what it should
*/
public static returntype putNumber(parametersIfAny)
{
add whatever code is required here in the method body
}
/**
* Resets so all numbers previously forgotten. This is
* dangerous since average is now undefined.
* This method needs to be completed. It needs:
* – a proper return type
* – a proper list of parameters (maybe empty)
* – code to actually do what it should
*/
public static returntype reset(parametersIfAny)
{
add whatever code is required here in the method body
}
/**
* Resets with the first number of a new series. This is
* safer reset since the average remains defined.
* This method needs to be completed. It needs:
* – a proper return type
* – a proper list of parameters (maybe empty)
* – code to actually do what it should
*/
public static returntype reset(parametersIfAny)
{
add whatever code is required here in the method body
}
/**
* This method needs to be completed. It needs:
* – a proper return type
* – a proper list of parameters (maybe empty)
* – code to actually do what it should
*/
public static returntype getSum(parametersIfAny)
{
add whatever code is required here in the method body
}
/**
* This method needs to be completed. It needs:
* – a proper return type
* – a proper list of parameters (maybe empty)
* – code to actually do what it should
*/
public static returntype getCount(parametersIfAny)
{
add whatever code is required here in the method body
}
/**
* This method needs to be completed. It needs:
* – a proper return type
* – a proper list of parameters (maybe empty)
*/
public static returntype getAverage(parametersIfAny)
{
/*
* Note: This body of this method “getAverage” has
* been provided in its entirety. You do NOT have
* to make any changes to it.
*/
return (
(double) sum / (double) count
);
}
} // class SummerStatic
Question 7
This exercise builds on the static version of the same exercise from last week. The idea is basically the same, we have series of methods to complete, but this time they are not static – i.e. they relate to object-level data.
This means that to actually use these methods and the data members they (should) access, an object needs to be created. As before, this part has actually already been done in the main
method, and it is useful to observe the differences between this week and last’s week’s required exercises.
In many ways, the result of this exercise will look very similar to the static version, but now we can have several BankAccount
s, with differing data.
To complete it, you have to provide return types, parameter lists and code for the following methods:
setInterestRate
getInterestRate
toString
You also have to complete two constructors. If you are unsure what a constructor is, or the syntax, check the Classes and Objects lesson, or talk to the teaching staff.
Question 7 Starter Code
/**
* This Object-Oriented” version of the “BankAccount” class
* is a simple introduction to Constructors /
* private data members / static vs. not static / and the
* “toString” method.
*
* SKELETON FOR LAB TEST.
*/
public class BankAccountOO {
public static double interestRate = 0.0;
// Note: the interest rate is “static”. That is, all
// bank accounts have the same interest rate.
/*
* The instance variables follow (i.e. not static). These
* are also called “fields” or “private data members”.
*/
public final int accountNumber;
public String accountName;
public double balance; // e.g. 1.27 means $1.27
public static void main (String[] args) {
BankAccountOO.setInterestRate(7.50);
System.out.println(“(Interest rate should be 7.50) Interest rate is ” + BankAccountOO.getInterestRate() + “.”);
BankAccountOO bankaccountoo = new BankAccountOO(99998888,”Jack”);
System.out.println(“So we created an account for Jack with zero dollars in his bank account under the account number 99998888.”);
System.out.println(“”);
System.out.println(“”);
System.out.println(“For the object created, the account number is: ” + bankaccountoo.accountNumber);
System.out.println(“For the object created, the account name is: ” + bankaccountoo.accountName);
System.out.println(“For the object created, the balance is: ” + bankaccountoo.balance);
System.out.println(“”);
System.out.println(“”);
System.out.println(“”);
System.out.println(“Now we use the constructor which takes three argumants including name, number and initial balance:”);
BankAccountOO bankaccountoo2 = new BankAccountOO(12345678,”Jane”,1000.99);
System.out.println(“So we created an account for Jane with 1000.99 dollars in her bank account under the account number 12345678”);
System.out.println(“”);
System.out.println(“”);
System.out.println(“For the second object created, the account number is: ” + bankaccountoo2.accountNumber);
System.out.println(“For the second object created, the account name is: ” + bankaccountoo2.accountName);
System.out.println(“For the second object created, the balance is: ” + bankaccountoo2.balance);
System.out.println(“”);
System.out.println(“”);
System.out.println(“”);
System.out.println(“Now we use the method toString to print it all in the same line”);
System.out.println(“First object: ” + bankaccountoo.toString());
System.out.println(“Second object: ” + bankaccountoo2.toString());
System.out.println(“”);
System.out.println(“”);
System.out.println(“Testing withdraw method on Jane’s account: Jane’s new balance after we withdraw 100.00 is ” + bankaccountoo2.withdraw(100.00));
}
/**
* The constructors now follow
*/
/**
* @param num number for the account
* @param name name of the account
*/
public returnTypeIfAny nameOfConstructor(parametersIfAny) {
add whatever code is required in the constructor body
}
/**
* @param num number for the account
* @param name name of the account
* @param bal opening balance
*/
public returnTypeIfAny nameOfConstructor(parametersIfAny) {
add whatever code is required in the constructor body
}
/*
* A number of the methods from the “static” version
* have been left out of this object-oriented version
* because you’ve already done that once.
*
* Most of those methods would appear in a complete
* version of this object-oriented version of the
* class, with the *ONLY* change being that the reserved
* word “static” would be deleted from the method
* header.
*
* The method setAccountNumber() would definitely NOT
* be copied across from the static version to this
* object-oriented version. That’s because each object
* (i.e. instance of BankAccount) represents a unique
* bank account. The account number set up by the
* constructor should never change again.
*
* The method setAccountName() MIGHT be copied across.
* That’s a business decision. Some banks might allow
* a customer to change their name. Other banks might
* only allow a name change by creating a new account.
* If the account name cannot be changed, then the
* declaration of private data member “accountName”
* should have the reserved word “final” added.
*
* The method withdraw() has been copied across
* because:
* (1) it was provided in its entirety in the
* “static” version of the class,
* (2) to provide an example that “static” is dropped
* from the header,
* (3) to provide a hint as to what changes might be
* needed for the other, incomplete methods.
*/
/**
* The withdrawal should be refused if the withdrawal
* would result in a negative balance.
*
* @param amount The amount to be withdrawn
*
* @return new balance or -1.0 if withdrawal refused
*/
public returntype withdraw(double amount) {
if ( balance >= amount ) {
balance = balance – amount;
return balance;
}
else
return -1.0;
}
/**
* Note that this is a static method. So a change to the
* interest rate changes the interest rate for all objects
* of this class.
*
* @param newInterest The new interest rate
*/
public static returntype setInterestRate(parametersIfAny) {
add whatever code is required here in the method body
}
/**
* Note that this is a static method.
*
* @return The interest rate
*/
public static returntype getInterestRate(parametersIfAny) {
add whatever code is required here in the method body
}
/**
* It is common practise to supply a “toString” method
* in an object-oriented class. In fact, if you don’t
* explicitly supply such a method, Java produces an
* implicit, simplistic “toString” method which produces
* a String like “BankAccountOO@1edd1f0”. The word before
* the “@” is the name of the class. The hexadecimal
* number after the “@” is called the objects “hash code”.
*
* Note: Method “toString” method is NOT “static”. It
* can’t be static, since the values in the data members
* may vary between objects of this class.
*
*@return The state of this “class instance” or “object”
*/
public returntype toString(parametersIfAny) {
return “accountNumber = ” + accountNumber +
” accountName = ” + accountName +
” balance = ” + balance;
}
} // class BankAccountOO
Question 8
As with the bank account exercises, here we revisit the Summer exercise and change from using static variables and methods to a mixture of static and non-static ones. As before, the immediate consequences to the written code are not dramatic, but the implied use is. Once this is complete, we can create multiple Summers, to keep as many sums as we care to, rather than only being able to retain a single sum in the static case. This exercise also includes a static variable and related static method that shows a more natural use of class level static data.
Your task here is to complete two constructors, and the methods getNumSummers and toString
Question 8 Starter Code
/**
* This Object-Oriented version of the “Summer” class
* is a simple introduction to constructors /
* private data members / static vs. not static / and the
* “toString” method.
*
* SKELETON FOR LAB TEST.
*
*
*/
public class SummerOO
{
static int numSummers = 0;
// The above variable is used to count the number of
// instances of the class SummerOO that have been created.
// (i.e. the number of objects that have been created.)
//
// Note: “numSummers” is declared as “static”. It is a
// variable that belongs to the class, not an
// individual object.
/*
* The instance variables follow (i.e. not static). These
* are also called “fields” or “data members”.
* Each object has its own copy of these variables.
*/
int sum; // sum of all integers received
int count; // number of integers received
/**
* The constructors now follow. There are two constructors.
* Both constructors have the same name, since all
* constructors always have the same name as the class.
* The constructors are distinguishable because one of the
* constructors requires zero parameters while the other
* constructor requires a single integer parameter.
*/
/**
* This is a “dangerous” constructor, since the average is
* undefined when the object is created.
*
* This constructor and the method reset()
* are similar. The differences are that:
* (1) The constructor can only be used once, when the
* object is created.
* (2) The method reset() can’t create an object, but it can
* be used whenever we like, as many times as we like,
* after the object has been created.
*/
public returnTypeIfAny nameOfConstructor()
{
add whatever code is required in the constructor body
}
/**
* This is a safer constructor, since the average is
* well defined when the object is created.
*
* This constructor and the method reset(int firstNumber)
* are similar. The differences are that:
* (1) The constructor can only be used once, when the
* object is created.
* (2) The method reset() can’t create an object, but can
* be used whenever we like, as many times as we like,
* after the object has been created.
*
* @param firstNumber The first number of a series
*/
public returnTypeIfAny nameOfConstructor(parameter)
{
add whatever code is required in the constructor body
}
/**
* Receives and processes a new number in the series.
*
* NOTE TO STUDENTS: When studying this code, experiment
* by adding “static” into “public void putNumber”.
* When you compile, you’ll get an error message …
*
* “non-static variable sum cannot be referenced from a
* static context”.
*
* In other words a “static” method (which belongs to the
* class, not an individuaual object), can’t access the
* variables in an object. This is for two reasons:
* (1) If we haven’t created ANY objects yet, then there is
* no variable “sum” to access!
* (2) If multiple objects (“instances”) of this
* class exist, then there are multiple versions of
* the “sum” variable, one version of “sum” in each
* object. The static method (which belongs to the
* class) cannot choose from the many versions of “sum”.
* The same applies to the variable “count”. The error
* message singled out “sum” because it occured before
* “count”.
*
* @param newNumber a new number in the series
*/
public /* not static! */ void putNumber(int newNumber)
{
// This method is complete. No changes are required to
// “putNumber” in the lab test.
sum = sum + newNumber; // could write “sum += newNumber”
count = count + 1; // could write “++count”
}
/*
* A number of the methods from the “static” version
* have been left out of this object-oriented version
* because you’ve already done that.
*
* All those methods would appear in a complete
* version of this object-oriented version of the
* class, with the *ONLY* change being that the reserved
* word “static” would be deleted from the method
* header.
*
* The method putNumber() has been copied across to support
* the experiment of adding “static” to its header,
*/
/**
* Note that this is a static method.
*
* @return The number of objects that have been created.
*/
public static returntype getNumSummers(parametersIfAny)
{
add whatever code is required here in the method body
}
/**
* It is common practise to supply a “toString” method
* in an object-oriented class. In fact, if you don’t
* explicitly supply such a method, Java produces an
* implicit, simplistic “toString” method which produces
* a String like “SummerOO@1edd1f0”. The word before
* the “@” is the name of the class. The hexadecimal
* number after the “@” is called the objects “hash code”.
*
* Note: Method “toString” method is NOT “static”. It
* can’t be static, since the values in the data members
* may vary between objects of this class.
*
*@return The state of this “class instance” / “object”
*/
public returntype toString(parametersIfAny)
{
return “sum = ” + sum + ” count = ” + count;
}
/**
* The main method is already complete, and you
* don’t need to change anything, but it won’t
* hurt anything if you do, as long as it still
* compiles.
*/
public static void main(String [] args)
{
SummerOO summer1 = new SummerOO();
// the above line used the zero parameter constructor.
summer1.putNumber(17);
summer1.putNumber(1);
System.out.println(summer1.toString());
// in the above line, the “.toString()” can be omitted,
// to give just …
// System.out.println(summer1);
//
// When the name of an object is given where a String
// is required (and println requires a String), Java
// automatically calls the “toString()” method of the
// object.
// Repeat for a second set of numbers
System.out.println();
SummerOO summer2 = new SummerOO(3);
// above line used the constructor that takes a parameter
summer2.putNumber(5);
summer2.putNumber(7);
System.out.println(summer2);
// in the above line, Java automatically calls the
// “toString()” method of the “summer2” object.
} // method main
} // class SummerOO
Question 9
The basic idea of a list in most programming languages is that of an array, but where elements can be added or removed (i.e. it can be resized).
In this exercise we’re going to take a step towards that idea and build a simplified version of a list backed by an array as the actually storage. That is, the class will store an array internally, but present a list-like interface to the outside world. The list will store ints
, to help keep things simple.
The skeleton starts with the basic class defined, and a single data member data
of type int[]
. It’s public
only to simplify testing, and final
to prevent one particular misstep in some of the tasks.
The tasks are then to complete the methods in the class, as given by their method signatures (more detail is given in the comments in the class as well):
MyArrayList()
– A constructor which will initialise the array and any other data members you add. The array should have length 6. Note that this is not the same as the size of the list, it just defines the maximum size. The list starts empty. The values stored in unused portions of the array are not important.boolean add(int)
– adds an element to the end of the list if there’s space. If there is, add it and returntrue
. If not, returnfalse
.int get(int)
– returns the value at the specified position.int size()
– return the current size of the list (not necessarily the length of the array, which is the maximum capacity of the list).boolean overwriteWith(int, int)
– replace the element at the specified position with the new element. If this is successful, returntrue
. If this is impossible, returnfalse
.void overwriteWith(MyArrayList)
– replace all the values in this list with the values from the other list. Note that this cannot fail, as the other list has the same capacity, so there is no return type.
Question 9 Starter Code
public class MyArrayList {
//The array to store the data.
public final int[] data;
//Constructor
public MyArrayList() {
this.data = null;
}
//Add element value to the end of the list
//return true if the element is added,
//false if there’s no space left
public boolean add(int value) {
return false;
}
//Return the element at position
//You don’t have to worry about
//positions less than 0 or greater
//than the array length – 1, but you
//do have to worry about positions that are
//after the end of the list. In that case
//return 0.
public int get(int position) {
return Integer.MIN_VALUE;
}
//Return the current size of the list
//Note that this may be different to
//the length of the array.
public int size() {
return -1;
}
//Replace the element at position with value
//Return true if the replacement happened,
//false otherwise.
public boolean overwriteWith(int position, int value) {
return false;
}
//Replace the data in the list with
//the elements of other. If other
//is smaller than the current list,
//the current list will get smaller.
public void overwriteWith(MyArrayList other) {
}
}

Get expert help for Data Structure Algorithms and many more. 24X7 help, plag free solution. Order online now!